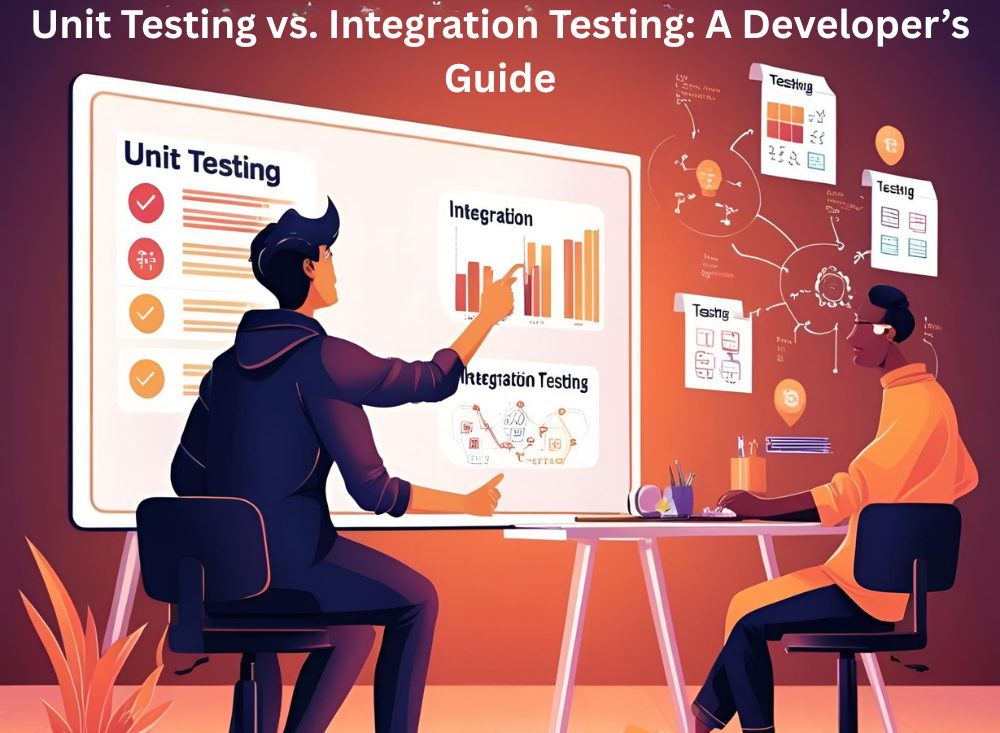
In the software development lifecycle, testing plays a vital role in ensuring that an application is functional, reliable, and free from defects. Among the various types of testing, unit testing and integration testing are two of the most fundamental and commonly used. While both serve distinct purposes, they are often misunderstood or used interchangeably.
This guide offers a comprehensive look at unit testing and integration testing, highlighting their differences, roles in the development process, benefits, and best practices. Whether you’re a new developer or looking to strengthen your testing strategies, understanding these concepts is essential for producing high-quality software.
What is Unit Testing?
Unit testing is the process of testing the smallest testable parts of an application, often referred to as “units.” In most cases, a unit is a single function, method, or class. The goal of unit testing is to verify that each unit performs as expected, in isolation from the rest of the application.
Key Characteristics of Unit Testing:
- Isolated: The unit is tested independently from other components.
- Fast: Since the scope is small, unit tests run quickly.
- Frequent: Developers often run unit tests during development to catch issues early.
- Focused: Each test is designed to validate a specific function or behavior.
For example, if you’re developing a calculator app, a unit test might check whether the add()
function correctly returns the sum of two numbers.
What is Integration Testing?
Integration testing takes a step beyond unit testing. Once individual units have been tested in isolation, integration testing verifies that they work together as a group. This process ensures that components interact correctly, data flows properly between modules, and combined behavior aligns with expectations.
Key Characteristics of Integration Testing:
- Combined Testing: Tests are written to check interactions between multiple modules.
- Realistic Scenarios: Integration tests mimic more real-world use cases than unit tests.
- Data Flow: These tests ensure that data passed between components is correct.
- Mid-Level Complexity: They sit between unit tests and full system tests in scope.
Continuing with the calculator example, integration testing might involve checking that the add()
function works when called through a user interface component or service layer, ensuring all pieces communicate correctly.
Core Differences Between Unit Testing and Integration Testing
Aspect | Unit Testing | Integration Testing |
---|---|---|
Scope | Single functions, methods, or classes | Multiple units or modules combined |
Focus | Internal logic and behavior | Interaction between components |
Isolation | Tests are isolated from other parts | Combines real or mocked dependencies |
Speed | Very fast | Slower due to more complexity |
Goal | Verify correctness of individual units | Verify cooperation between units |
When Used | During development, frequently | After unit testing, before full system testing |
Maintenance | Easier to maintain | Harder due to interdependencies |
Why Both Are Important
Relying solely on either unit tests or integration tests is insufficient for building reliable systems. Each type of test covers a different part of the risk landscape:
- Unit tests catch bugs in individual components early, making them easy to fix.
- Integration tests catch bugs caused by incorrect assumptions about how different components interact.
Together, they provide a strong safety net that helps ensure not only that each piece of code works, but also that they work well together.
Common Challenges in Unit and Integration Testing
Unit Testing Challenges:
- Mocking Dependencies: Isolating code sometimes requires replacing real dependencies with fake ones, which can be tricky.
- Over-Isolation: Excessive focus on isolated units might ignore how they’re used in real applications.
- Testing Private Logic: It’s sometimes difficult to test non-public parts of the code without breaking encapsulation.
Integration Testing Challenges:
- Flakiness: Because they involve multiple systems, integration tests are more prone to failures due to timing, configuration, or environment issues.
- Setup Complexity: Creating test environments that mimic production scenarios takes time and effort.
- Debugging Failures: When an integration test fails, it’s often less clear which component is at fault.
Best Practices for Unit Testing
- Write Small, Focused Tests: Each test should validate a specific behavior.
- Name Tests Clearly: Test names should describe what they verify.
- Test Edge Cases: Don’t just check the “happy path.” Handle unusual or invalid inputs.
- Maintain High Test Coverage: While 100% coverage isn’t mandatory, strive for thorough coverage of critical paths.
- Run Tests Often: Integrate testing into your daily workflow.
Best Practices for Integration Testing
- Test Important Interactions: Focus on high-value connections like data flows, APIs, or shared resources.
- Use Realistic Data: Your tests should simulate real user scenarios as closely as possible.
- Isolate External Systems: When possible, avoid reliance on external systems (e.g., third-party services) to reduce flakiness.
- Monitor Performance: Integration tests can reveal not just logic bugs but also performance bottlenecks.
- Automate Execution: Include integration tests in your regular test suites to catch issues early.
When to Write Unit vs. Integration Tests
Knowing when to use unit or integration tests depends on the stage of development and what you’re trying to verify.
- Early in development: Unit tests are ideal for building confidence in individual components.
- After modules are completed: Use integration tests to ensure the components work together.
- When fixing bugs: Write unit tests to confirm the specific bug is fixed and doesn’t reoccur. Follow up with integration tests if the bug involved multiple modules.
- Before releasing software: Integration tests play a critical role in acceptance testing and regression prevention.
Test Pyramid: Balancing Test Types
A commonly used model in software testing is the test pyramid, which suggests a layered approach to testing:
- Base Layer – Unit Tests: Form the largest portion. Fast, frequent, and detailed.
- Middle Layer – Integration Tests: Fewer in number but broader in scope.
- Top Layer – End-to-End or System Tests: Highest-level tests that simulate full user behavior.
The pyramid encourages a broad base of fast, reliable unit tests, supported by more selective integration tests, ensuring optimal test efficiency and effectiveness.
Conclusion
Both unit testing and integration testing are crucial to building robust, scalable software. While unit testing provides the foundation for quality at the component level, integration testing ensures that everything works seamlessly together. Ignoring one in favor of the other creates blind spots and increases the risk of failure as the software grows.
By understanding the strengths and limitations of each, developers can design a balanced testing strategy that catches bugs early, simplifies maintenance, and boosts confidence in the software’s performance. In today’s fast-paced development environments, where change is constant and expectations are high, investing in thoughtful, well-structured tests is not just good practice—it’s a necessity.