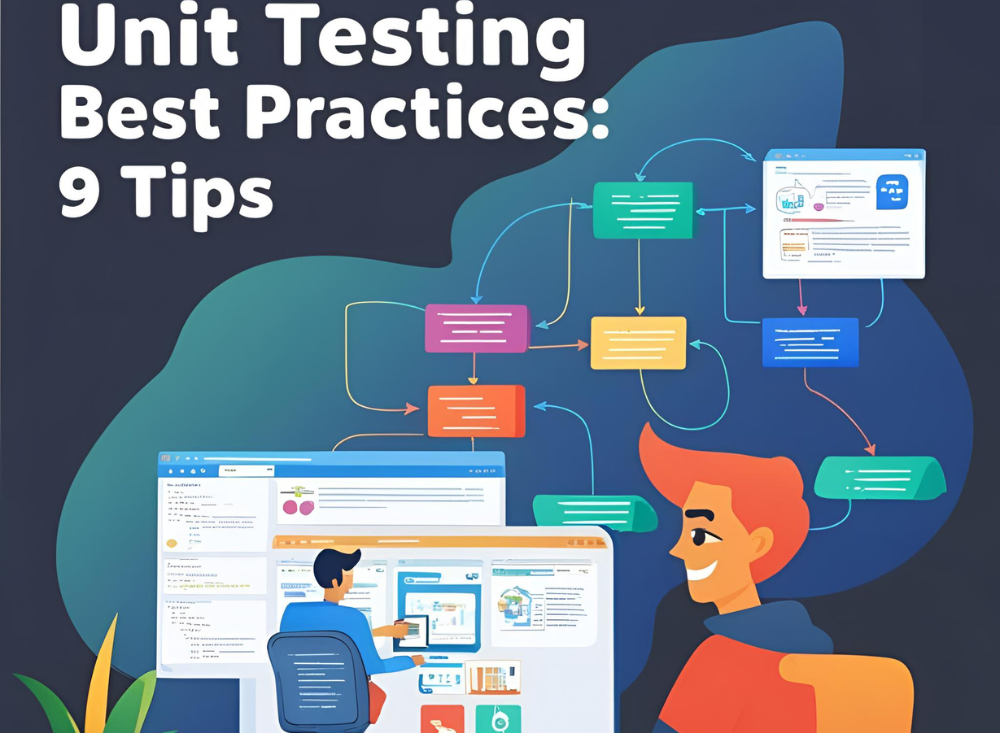
Unit testing is a fundamental practice in software development that involves testing individual components or units of code to ensure they function as intended. When done correctly, unit testing improves code quality, reduces bugs, and makes the development process more efficient. However, writing effective unit tests requires more than just creating test cases — it requires following best practices to ensure your tests are reliable, maintainable, and valuable. In this article, we’ll explore nine unit testing best practices to help you do it right.1. Write Tests Before Writing Code (Test-Driven Development)
Test-Driven Development (TDD) is a methodology where you write tests before writing the actual code. This approach ensures that your code is designed to meet specific requirements and encourages you to think about edge cases and potential issues upfront.
Why It Matters:
- Forces you to define clear requirements before coding.
- Reduces the likelihood of writing unnecessary or overly complex code.
- Ensures that your code is testable from the start.
2.Keep Tests Small and Focused
Unit tests should be small and focus on testing a single piece of functionality. Avoid writing tests that cover multiple scenarios or dependencies, as this can make them difficult to understand and maintain.
Why It Matters:
- Makes tests easier to read and debug.
- Ensures that failures are specific and actionable.
- Reduces the risk of tests becoming brittle or unreliable.
3.Use Descriptive Test Names
The name of your test should clearly describe what it is testing and the expected outcome. A well-named test serves as documentation and makes it easier to identify issues when a test fails.
Why It Matters:
- Improves readability and maintainability.
- Helps other developers understand the purpose of the test.
- Makes it easier to pinpoint the cause of a failure.
Example:
- Instead of
testCalculate()
, usetestCalculate_DiscountAppliedCorrectly()
.
4.Follow the Arrange-Act-Assert Pattern
The Arrange-Act-Assert (AAA) pattern is a structured way to write unit tests. It divides the test into three clear sections:
- Arrange: Set up the necessary preconditions and inputs.
- Act: Execute the code being tested.
- Assert: Verify that the output matches the expected result.
Why It Matters:
- Makes tests more organized and easier to follow.
- Ensures that each test has a clear purpose and outcome.
- Reduces the risk of missing critical steps.
5.Test Edge Cases and Error Conditions
While it’s important to test the “happy path” (i.e., the expected behavior), don’t forget to test edge cases and error conditions. These include invalid inputs, boundary values, and unexpected scenarios.
Why It Matters:
- Ensures your code handles unexpected situations gracefully.
- Reduces the likelihood of bugs in production.
- Improves the robustness of your application.
Example:
- Test how your function handles null values, empty strings, or out-of-range inputs.
6.Avoid Testing Implementation Details
Unit tests should focus on the behavior of the code, not its internal implementation. Testing implementation details can make your tests brittle, meaning they break easily when the code is refactored, even if the behavior remains the same.
Why It Matters:
- Makes tests more resilient to changes in the codebase.
- Encourages better design by focusing on outcomes rather than specifics.
- Reduces the maintenance burden of updating tests.
7.Keep Tests Independent
Each unit test should be independent of other tests. Avoid relying on shared state or the outcome of previous tests, as this can lead to unpredictable results and make debugging difficult.
Why It Matters:
- Ensures that tests can be run in any order.
- Makes it easier to isolate and fix failures.
- Improves the reliability of your test suite.
8.Use Mocks and Stubs Wisely
Mocks and stubs are used to simulate dependencies or external systems in your tests. While they are useful, overusing them can make your tests less realistic and harder to maintain.
Why It Matters:
- Ensures that your tests focus on the unit being tested, not its dependencies.
- Reduces the complexity of setting up test environments.
- Avoids creating tests that are too tightly coupled to specific implementations.
Best Practice:
- Use mocks and stubs only when necessary, such as for external APIs or databases.
9.Regularly Review and Refactor Tests
Just like your production code, your tests should be reviewed and refactored regularly. Over time, tests can become outdated, redundant, or overly complex, reducing their effectiveness.
Why It Matters:
- Keeps your test suite maintainable and relevant.
- Ensures that tests continue to provide value as the codebase evolves.
- Identifies opportunities to improve test coverage or efficiency.
How to Do It:
- Periodically review tests for readability and relevance.
- Remove redundant or outdated tests.
- Refactor tests to improve clarity and reduce duplication.
Conclusion
Unit testing is a powerful practice that can significantly improve the quality and reliability of your code. By following these nine best practices, you can ensure that your unit tests are effective, maintainable, and valuable. Remember, the goal of unit testing is not just to catch bugs but to create a safety net that allows you to refactor and improve your code with confidence. Invest time in writing high-quality tests, and you’ll reap the benefits throughout the development lifecycle.