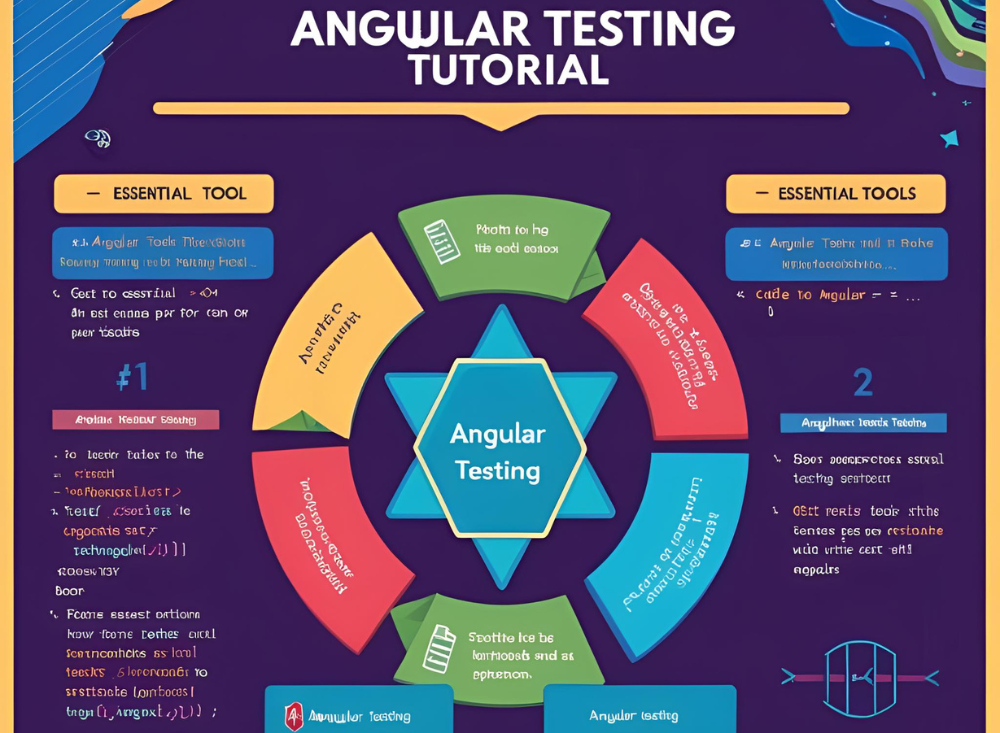
Testing is a critical aspect of building robust and maintainable Angular applications. It ensures that your code works as expected, catches bugs early, and provides confidence when making changes. Angular provides a powerful testing framework that supports various types of tests, including unit tests, integration tests, and end-to-end (E2E) tests. In this tutorial, we’ll explore what Angular testing is, how it works, and how to get started with testing your Angular applications.
Angular Testing: How It Works?
Angular testing revolves around verifying the functionality, behavior, and performance of your application. The Angular framework is designed with testability in mind, providing built-in utilities and libraries to simplify the testing process. Angular applications are typically tested at three levels:
- Unit Tests: Focus on individual components, services, or functions.
- Integration Tests: Verify the interaction between multiple components or services.
- End-to-End (E2E) Tests: Simulate real user interactions with the application.
These tests form the Testing Pyramid, a model that emphasizes the importance of having a solid foundation of unit tests, supported by integration tests, and a smaller number of E2E tests.
The Testing Pyramid
1.Unit Tests
Unit tests are the foundation of the testing pyramid. They focus on testing individual units of code in isolation, such as a single component, service, or function. Unit tests are fast, easy to write, and provide immediate feedback.
Why Unit Tests Matter:
- Catch bugs early in the development process.
- Ensure individual components work as expected.
- Provide a safety net for refactoring.
2.Integration Tests
Integration tests verify how multiple units of code work together. For example, you might test how a component interacts with a service or how multiple components interact within a module.
Why Integration Tests Matter:
- Ensure that different parts of the application work together correctly.
- Catch issues that unit tests might miss, such as communication errors between components.
- Provide confidence in the overall functionality of the application.
3.End-to-End Tests
End-to-end (E2E) tests simulate real user interactions with the application. They test the application as a whole, from the user interface to the backend.
Why E2E Tests Matter:
- Validate the complete user experience.
- Catch issues related to navigation, data flow, and UI interactions.
- Ensure the application works as expected in a real-world scenario.
How to Start Angular Testing
1.Set Up Your Testing Environment
Angular provides a testing environment out of the box, so you don’t need to configure much to get started. Ensure your project is set up with the necessary testing libraries and configurations.
2.Write Unit Tests
Start by writing unit tests for individual components, services, and pipes. Use Angular testing utilities to create isolated test environments for your units of code.
Steps for Writing Unit Tests:
- Create a test file for the component or service (e.g.,
my-component.component.spec.ts
). - Use
TestBed
to configure the testing module and declare the component or service. - Write test cases to verify the behavior of the unit.
Example:
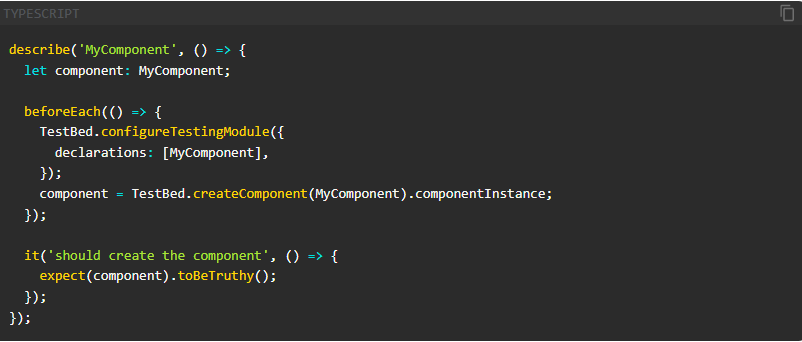
3.Serve Data for Integration Tests
Integration tests often require data to simulate real-world scenarios. Use Angular’s HttpClientTestingModule
to mock HTTP requests and provide test data.
Steps for Serving Data:
- Import
HttpClientTestingModule
into your testing module. - Use
HttpTestingController
to mock HTTP responses. - Write test cases to verify how components interact with services.
4.Set Up Integration Tests
Integration tests involve testing the interaction between multiple components or services. Use Angular’s testing utilities to create a realistic environment for these tests.
Steps for Setting Up Integration Tests:
- Configure the testing module with all necessary components and services.
- Use
TestBed
to create the test environment. - Write test cases to verify the interaction between components or services.
Example:
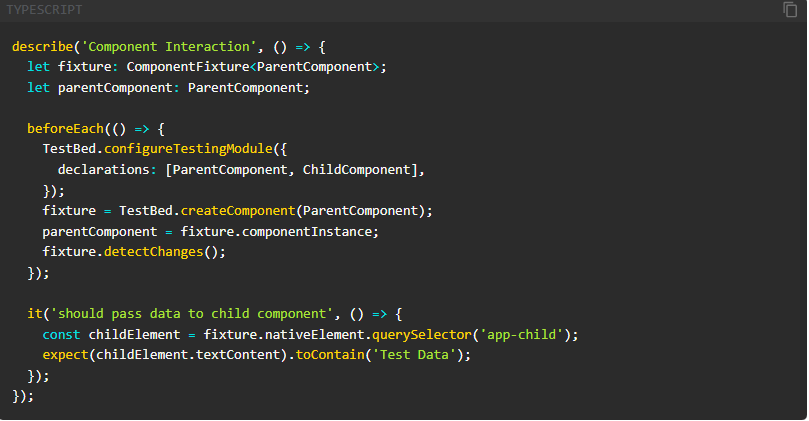
5.Write End-to-End Tests
End-to-end tests simulate real user interactions with the application. Use Angular’s E2E testing framework to write tests that navigate the application, interact with elements, and verify outcomes.
Steps for Writing E2E Tests:
- Create a test file (e.g.,
app.e2e-spec.ts
). - Write test cases to simulate user actions, such as clicking buttons or filling out forms.
- Use assertions to verify the expected outcomes.
Example:
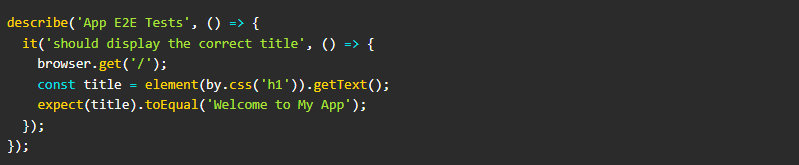
Conclusion
Testing is an essential part of building reliable and maintainable Angular applications. By following the testing pyramid and writing unit tests, integration tests, and end-to-end tests, you can ensure that your application works as expected and delivers a great user experience. Start small with unit tests, gradually move to integration tests, and use E2E tests to validate the complete application. With Angular built-in testing utilities, you have everything you need to get started. Happy testing!